Construct
Angular
Forms the
Right Way
Build Angular custom form components efficiently
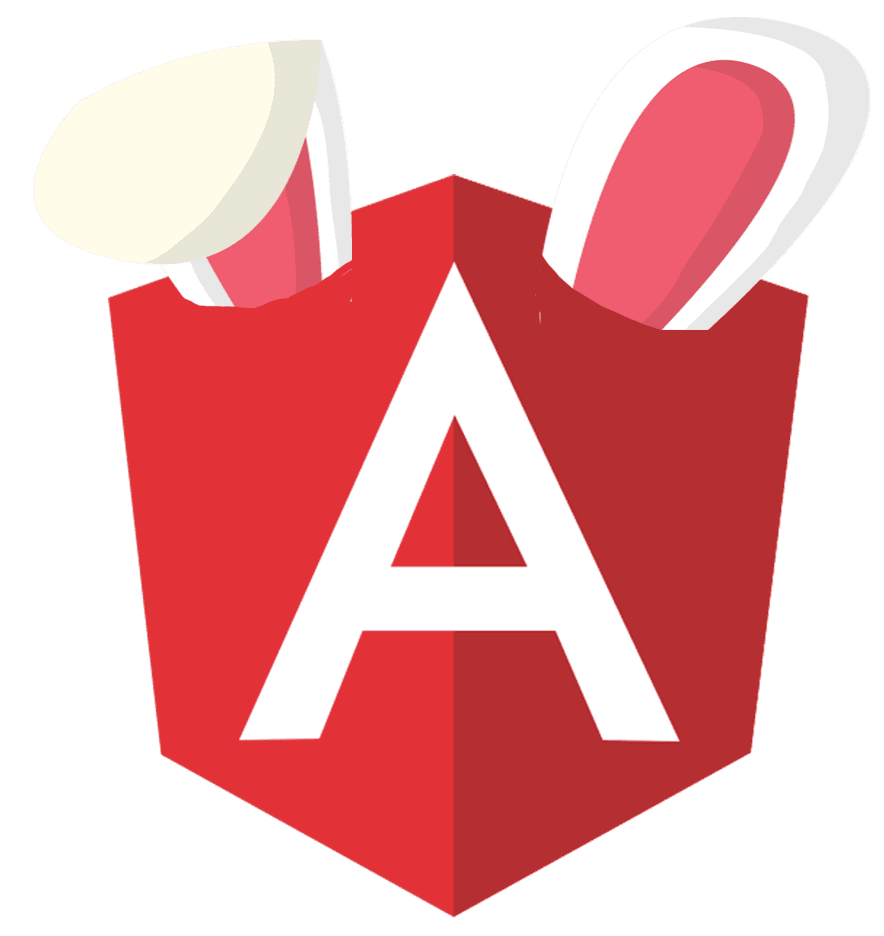
Feel the Difference
Transform Your Custom Form Components
to
1import { Component } from '@angular/core';
2import { ControlValueAccessor, NG_VALUE_ACCESSOR } from '@angular/forms';
3
4@Component({
5 selector: 'app-file-upload',
6 template: './FileUploadComponent.html',
7 providers: [
8 {
9 provide: NG_VALUE_ACCESSOR,
10 multi: true,
11 useExisting: hadarComp2
12 }
13 ]
14})
15
16export class hadarComp2 implements ControlValueAccessor {
17
18 onChange = (value: string) => { }
19
20 onTouched = () => { }
21
22 value: string = ''
23
24 disabled: boolean = false
25
26 onFileSelected(event) {
27 const file = event.target.files[0];
28 if (file) {
29 this.value = file.name;
30 console.log(this.value);
31 this.onChange(this.value);
32 }
33 }
34
35 onClick(fileUpload: HTMLInputElement) {
36 this.onTouched();
37 fileUpload.click();
38 }
39
40 writeValue(value: any) {
41 this.value = value
42 }
43
44 registerOnChange(onChange: any) {
45 this.onChange = onChange
46 }
47
48 registerOnTouched(onTouched: any){
49 this.onTouched = onTouched
50 }
51
52 setDisabledState(disabled: boolean): void {
53 this.disabled = disabled
54 }
55}

How To Use Our Package
3 Simple Steps to Solving Your Form Building Problems
Step One
NPM Install @AngoraForms
Step Two
Consult our docs to correctly configure your Angular application
Step Three
Concisely write your custom form components all in one file!